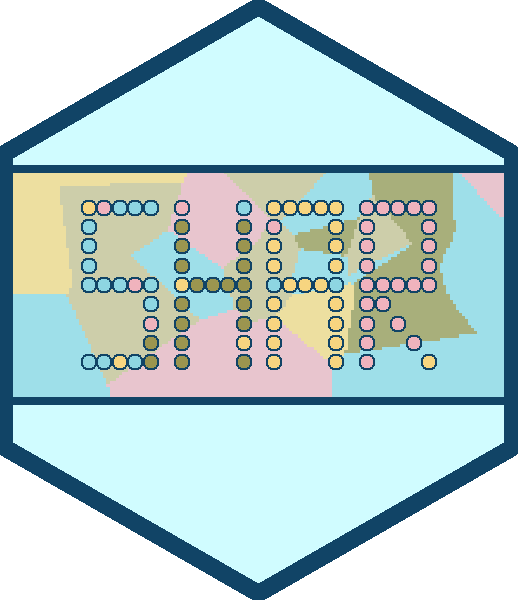
How to reconstruct multiple patterns
Maximilian H.K. Hesselbarth
2023-12-15
Source:vignettes/articles/reconstruct_multiple_patterns.Rmd
reconstruct_multiple_patterns.Rmd
In case you want to reconstruct several patterns at once (e.g. for
different points in time if repeated censuses are available), you can
use the following code. Please be aware that the maximum number of
iterations was set to max_runs = 10
to keep the
computational time low for this example. For an applied case, this value
should be increased.
In case you want to only create the spatial characteristics, this is
straightforward using lapply()
.
# create list with patterns
list_pattern <- list(species_a, species_b)
# reconstruct all patterns in list
result <- lapply(list_pattern, function(x) reconstruct_pattern(pattern = x, n_random = 3,
max_runs = 10, verbose = FALSE))
The result will be a nested list including all m randomization (including the observed pattern) of the n provided input patterns.
# get mean energy
lapply(result, function(x) calculate_energy(pattern = x,
verbose = FALSE))
#> [[1]]
#> randomized_1 randomized_2 randomized_3
#> 0.14265579 0.11153049 0.09649081
#>
#> [[2]]
#> randomized_1 randomized_2 randomized_3
#> 0.2977567 0.3698121 0.4142496
Another possible would be to first reconstruct n times the spatial characteristics and afterwards reconstruct the marks m times for each of the n spatial reconstructions.
Firstly, reconstruct only the spatial characteristics n
times. The observed pattern is not needed in this case, so you can put
return_input = FALSE
.
# reconstruct spatial strucutre
reconstructed_pattern <- reconstruct_pattern(species_a, n_random = 3,
max_runs = 10, return_input = FALSE,
verbose = FALSE)
Secondly, to reconstruct the (numeric) marks of the observed pattern
for each of the spatially reconstructed patterns, just use
lapply()
in combination with
reconstruct_pattern_marks()
.
# get only selected marks of input (numeric marks)
species_a_marks <- subset(species_a, select = dbh)
# reconstruct marks 3 times for each input pattern
result_marks <- lapply(reconstructed_pattern$randomized,
function(x) reconstruct_pattern_marks(pattern = x,
marked_pattern = species_a_marks,
max_runs = 10,
n_random = 3, verbose = FALSE))
Again, the result is a nested list with the same dimensions as provided input patterns and reconstructions.
# get energy
lapply(result_marks, function(x) calculate_energy(pattern = x,
verbose = FALSE))
#> $randomized_1
#> randomized_1 randomized_2 randomized_3
#> 0.04731652 0.06264170 0.05364442
#>
#> $randomized_2
#> randomized_1 randomized_2 randomized_3
#> 0.03790957 0.04341131 0.04551169
#>
#> $randomized_3
#> randomized_1 randomized_2 randomized_3
#> 0.04960646 0.05829529 0.07140692