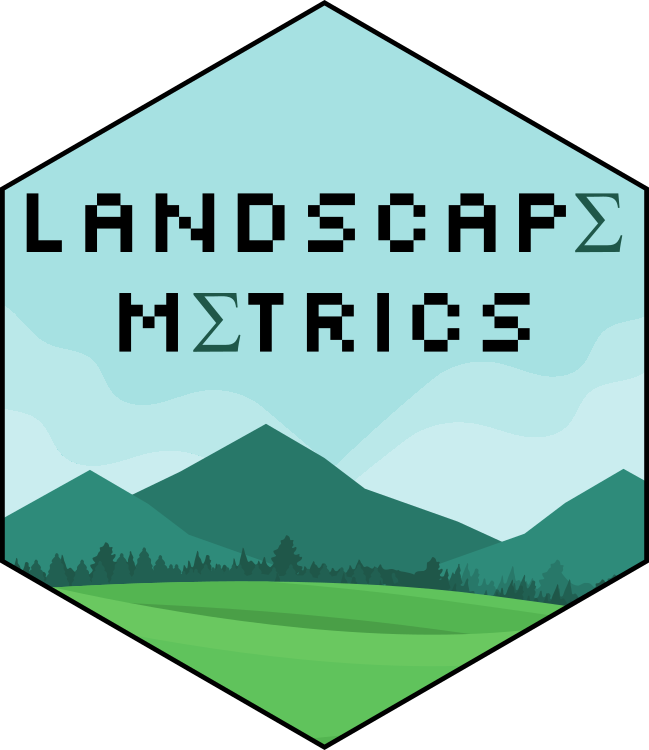
Landscape distribution statistics
2025-02-11
Source:vignettes/articles/landscape_distribution_statistics.Rmd
landscape_distribution_statistics.Rmd
Landscape distribution statistics summarise the properties of all
patches belonging to the same class i or of all patches in the
landscape together (McGarigal et al. 2012). This includes, for example,
the mean, the coefficient of variation, or the standard deviation of a
certain metric. These measures are implemented in the
landscapemetrics package and are indicated by the
corresponding suffixes (_mn
, _cv
,
_sd
). However, other distribution statistics also might be
of interest. Even though they are not implemented in
landscapemetrics, R allows to calculate them
straightforwardly.
library(landscapemetrics)
library(terra)
library(dplyr)
# internal data needs to be read
landscape <- terra::rast(landscapemetrics::landscape)
Implemented distribution statistics
The already implemented distribution statistics on class- and
landscape-level are indicated by the corresponding suffixes
(_mn
= mean, _cv
= Coefficient of variation ,
_sd
= Standard deviation) and can be calculated
directly.
# calculate mean shape index on class level
mean_shape_c <- lsm_c_shape_mn(landscape)
# calculate standard deviation of shape index on landscape level
sd_shape_l <- lsm_l_shape_sd(landscape)
Area-weighted mean
First, we want to calculate the metric of interest on the patch level. Additionally, we want the patch area of each patch.
# calculate required metric for each patch (e.g. lsm_p_shape)
metric_patch <- lsm_p_shape(landscape)
metric_patch
## # A tibble: 28 × 6
## layer level class id metric value
## <int> <chr> <int> <int> <chr> <dbl>
## 1 1 patch 1 1 shape 1
## 2 1 patch 1 2 shape 1.34
## 3 1 patch 1 3 shape 1.77
## 4 1 patch 1 4 shape 1
## 5 1 patch 1 5 shape 1
## 6 1 patch 1 6 shape 1.90
## 7 1 patch 1 7 shape 1.38
## 8 1 patch 1 8 shape 1.44
## 9 1 patch 1 9 shape 1.12
## 10 1 patch 2 10 shape 1.63
## # ℹ 18 more rows
# calculate area for each patch
area_patch <- lsm_p_area(landscape)
area_patch
## # A tibble: 28 × 6
## layer level class id metric value
## <int> <chr> <int> <int> <chr> <dbl>
## 1 1 patch 1 1 area 0.0001
## 2 1 patch 1 2 area 0.0005
## 3 1 patch 1 3 area 0.0072
## 4 1 patch 1 4 area 0.0001
## 5 1 patch 1 5 area 0.0001
## 6 1 patch 1 6 area 0.008
## 7 1 patch 1 7 area 0.0016
## 8 1 patch 1 8 area 0.0003
## 9 1 patch 1 9 area 0.0005
## 10 1 patch 2 10 area 0.0034
## # ℹ 18 more rows
Now, we should join the metric value with the area of each patch,
multiply the two values, and calculate the area-weighted mean. To
calculate the area-weighted mean on landscape-level, comment out the
dplyr::group_by()
line.
# calculate weighted mean
metric_wght_mean <- dplyr::left_join(x = metric_patch, y = area_patch,
by = c("layer", "level", "class", "id")) |>
dplyr::mutate(value.w = value.x * value.y) |>
dplyr::group_by(class) |>
dplyr::summarise(value.am = sum(value.w) / sum(value.y))
metric_wght_mean
## # A tibble: 3 × 2
## class value.am
## <int> <dbl>
## 1 1 1.74
## 2 2 1.46
## 3 3 1.97
Range
The range equals to the minimum value subtracted from the maximum
value of the metric of interest for each patch (McGarigal et al. 2012).
This can be calculated using the min()/max()
function or
the range()
function.
# class level
metric_range_c <- dplyr::group_by(metric_patch, class) |>
dplyr::summarise(range = max(value) - min(value))
metric_range_c
## # A tibble: 3 × 2
## class range
## <int> <dbl>
## 1 1 0.901
## 2 2 0.633
## 3 3 1.02
## [1] 1.351802
Median
The median equals to the value that divides all (ordered) values into
two equal groups. There is a base R function available,
median()
, that can be applied to values grouped by class or
all values (landscape-level).
# class level
metric_md_c <- dplyr::group_by(metric_patch, class) |>
dplyr::summarise(median = median(value))
metric_md_c
## # A tibble: 3 × 2
## class median
## <int> <dbl>
## 1 1 1.34
## 2 2 1.06
## 3 3 1.65
# landscape level
metric_md_l <- median(metric_patch$value)
metric_md_l
## [1] 1.337487
References
- McGarigal K., SA Cushman, and E Ene. 2023. FRAGSTATS v4: Spatial Pattern Analysis Program for Categorical Maps. Computer software program produced by the authors; available at the following web site: https://www.fragstats.org